wustoj-c++
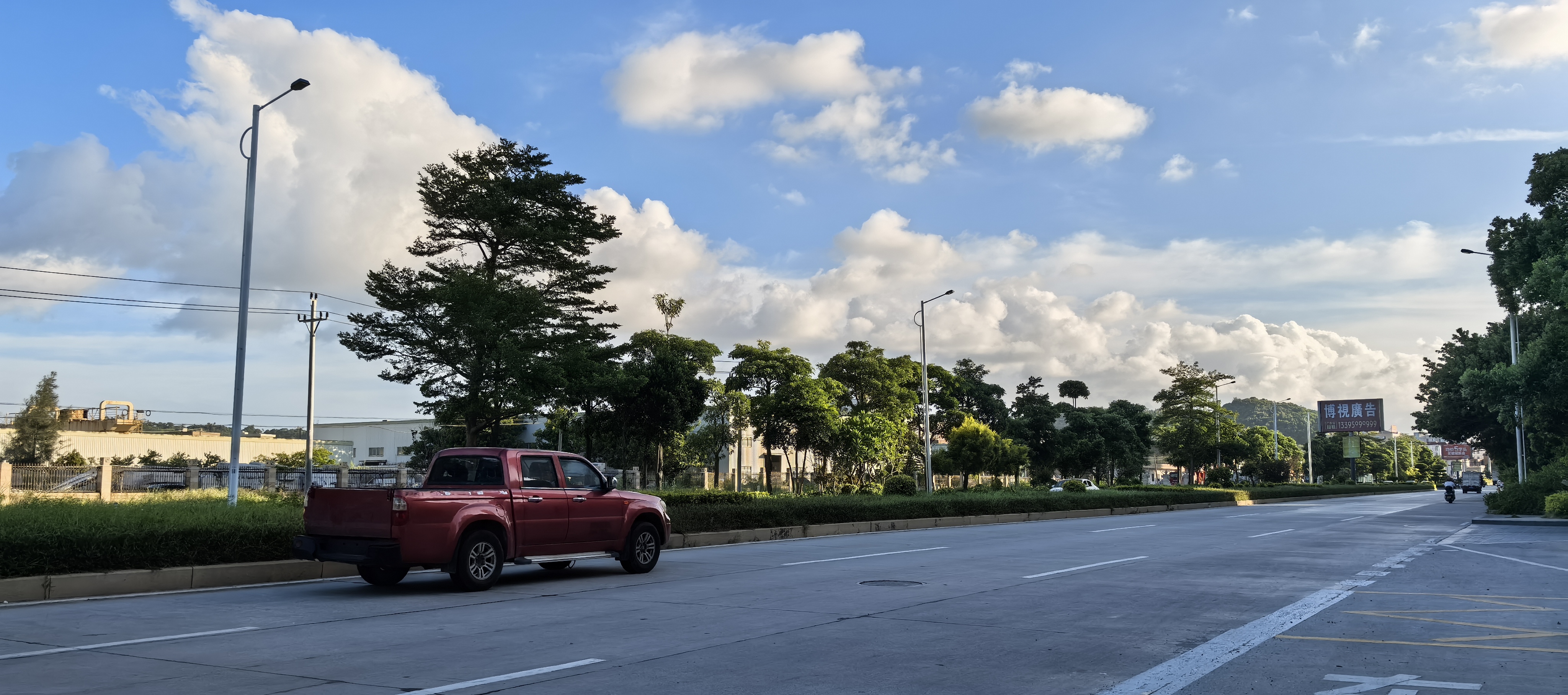
wustoj-c++
scandi由于 wustoj 属于闭源项目,无法看到他人提交代码,故在此记录个人 ac 代码,以便大家共同交流希望你不是直接 CV 大法! 本博文将随个人进度持续更新。
wustoj 不知道是谁在负责维护,我联系不到,部分题有出现错误,有题目可能是笔误,有测试数据可能有错误。我站在我的角度客观分析题目,如仍有错误,望指正!
代码旨在直接解决题目要求,代码格式不规范请谅解
1.类和对象
c++1001 时钟类 Clock 的设计
c++
1 |
|
c++1002 点类 Point 的设计
c++
1 |
|
c++1003 圆类 Circle 的设计
c++
1 |
|
c++.1004 矩形类 Rectangle 的设计
c++
1 |
|
c++.1005 复数类 Complex 的设计
这道题个人认为测试数据有问题!下面给出两段代码,第一段是能够 ac 这题的代码(不过其实是错误的,当虚部为 1 或者-1 时,会表现出 1i 或者-1i。)。第二段代码则是我认为的正解!(估计是测试数据出错了
c++
1 |
|
c++
1 |
|
c++.1006 日期类 Date 的设计
c++
1 |
|
c++.1007 学生类 Student 的设计
c++
1 |
|
c++.1008 线段类 Line 的设计
c++
1 |
|
c++.1009 国家类 Country 的设计
c++
1 |
|
c++.1010 长期存款类 Fixed_Deposit 的设计
c++
1 |
|
c++.1011 书类 Book 的设计
c++
1 |
|
c++.1012 建筑物类 building 的设计
c++
1 |
|
c++.1013 课程类 Course 的设计
本题的提供代码中,id 的数据类型是 double,这里题目笔误,id 的数据类型应该是 int(自行修改。
c++
1 |
|
c++.1014 实验室类 Laboratory 的设计
c++
1 |
|
c++.1015 个人 Person 类的设计
c++
1 |
|
2.运算符重载
c++.2001 时间类运算符重载
c++
1 |
|
c++.2002 日期类运算符重载
c++
1 |
|
c++.2003 复数类运算符重载
c++
1 |
|
c++.2004 学生类运算符重载
c++
1 |
|
c++.2005 短整数类运算符重载
本题在定义除法运算符号时,题目没有说明当 除数 为 0 时,应该返回什么来表明无意义计算。但这是应该注意的事情!
c++
1 |
|
c++.2006 个人 Person 类运算符重载
c++
1 |
|
c++.2007 圆类运算符重载
c++
1 |
|
c++.2008 分数类运算符重载
注意!本题下方有提示 “分数的输出要求。。。最简形式” 这里即使分子为 0 时,输出仍然不可省略分母部分。。。即使分子等于分母,或者分子分母互为相反数,也只能输出 1/1 或者 -1/1,而不能输出 1 或者 -1。
这题被防了很久。。。我很无语
c++
1 |
|
c++,2009 整型动态数组类及运算符重载
c++
1 |
|
c++.2010 分数类运算符重载(2)
c++
1 |
|
c++.2011 圆类 运算符重载(2)
c++
1 |
|
c++.2012 矩形类运算符重载
c++
1 |
|
c++.2013 日期类运算符重载(2)
这里的题干。。。。。。。。。
c++
1 |
|
c++.2014 时间类运算符重载(2)
c++
1 |
|
c++.2015 字符串 string 运算符重载
c++
1 |
|
c++.2016 字符串 string 运算符重载(2)
c++
1 |
|
c++.2017 矩形类运算符重载(2)
c++
1 |
|
c++.2018 复数类运算符重载(2)
c++
1 |
|
c++.2019 学生类运算符重载(2)
c++
1 |
|
c++.2020 个人 Person 类运算符重载(2)
c++
1 |
|
c++.CSTRING 类的设计
c++
1 |
|
c++.3001 日期类(由时间类派生)
c++
1 |
|
c++
1 |
c++
1 |
评论
匿名评论隐私政策